This week I stepped into serial communication between computer and micro-controller (Arduino Nano 33 IoT).
What is Serial Communication?
This is a process of sending data one bit at a time, sequentially over a computer channel or computer bus. Asynchronous means that the two computers that are communicating are each keeping track of time independently.
What does “9600” mean in Serial.println(9600)?
9600 means two devices are to exchange data at a rate of 9600 bits per second (9600 baud). The transmit (sometimes called TX) and the receive (sometimes called RX) are relative: my transmit is connected to your receive, and vice versa.
Lab Part
I followed the lab “Intro. to Asynchronous Serial Communication“

But ,
screen /dev/cu.usbmodem14101
gives an error “could not find a pty”. I solved it referencing to this answer:
In my case, there were no other “screen” processes running, but simply unplugging the device from the computer (i.e., USB to serial adapter) then plugging back in again cleared the issue.
Disconnect from the interactive window:
control-a control-\
One important takeaway: only one program can control a serial port at a time. When not using a given program, close the serial port.
Data: ASCII vs. Binary
Two ways of communication between two devices: 1. as a series of bits (raw binary data); 2. as a series of alphanumeric characters (ASCII-encoded data).
void setup() {
// start serial port at 9600 bps:
Serial.begin(9600);
}
void loop() {
int analogValue = analogRead(A0);
Serial.println(analogValue); // output a ASCII-encoded charater
Serial.write(analogValue); // output binary values
}
Arduino is sending out the text string where each character encoded as a single byte, which can be interpreted as ASCII code in some monitor. Serial.println() formats the value it prints as an ASCII-encoded decimal number, with a linefeed at a carriage return at the end. The serial monitor, receiving the data, assumes it should show you the ASCII character corresponding to each byte it receives.
Serial.write() command doesn’t format the bytes as ASCII characters. It sends out the binary value of the sensor reading.
For example, if analogValue is 32, then,
Serial.println(analogValue) results in “32” with a linefeed and carriage return;
Serial.write(analogValue) results in ” ” the space character, which has the ASCII value 32.
ASCII: ASCII code is the numerical representation of a character such as ‘a’ or ‘@’ or an action of some sort.
Binary: 0, 1
1 byte = 8 bits = 28
analog-to-digital converter (ADC) reads the input with a resolution of 10 bits
Flow Control
This is part that is quite related to my other class “Live Web”. It is important to apply call-and-response when sometimes we do not want the program to be overloaded. Therefore, when the sender sends faster than the receiver can read, we need to apply flow control.
Example:
void setup() {
Serial.begin(9600);
// sends out a message until it gets a byte of data from the remote computer:
while (Serial.available() <= 0) {
Serial.println("hello"); // send a starting message
delay(300); // wait 1/3 second
}
}
void loop() {
if (Serial.available()) {
// read the incoming byte:
int inByte = Serial.read();
// read the sensor:
sensorValue = analogRead(A0);
// print the results:
Serial.print(sensorValue);
Serial.print(",");
// read the sensor:
sensorValue = analogRead(A1);
// print the results:
Serial.print(sensorValue);
Serial.print(",");
// read the sensor:
sensorValue = digitalRead(switchPin);
// print the results:
Serial.println(sensorValue);
}
}
Getting into P5.js
When I installed p5.serialcontrol API, I encountered the same issue when I installed coolTerm on my computer. I found it could be solved easily by change the security setting in system preference.
Obtain serial ports
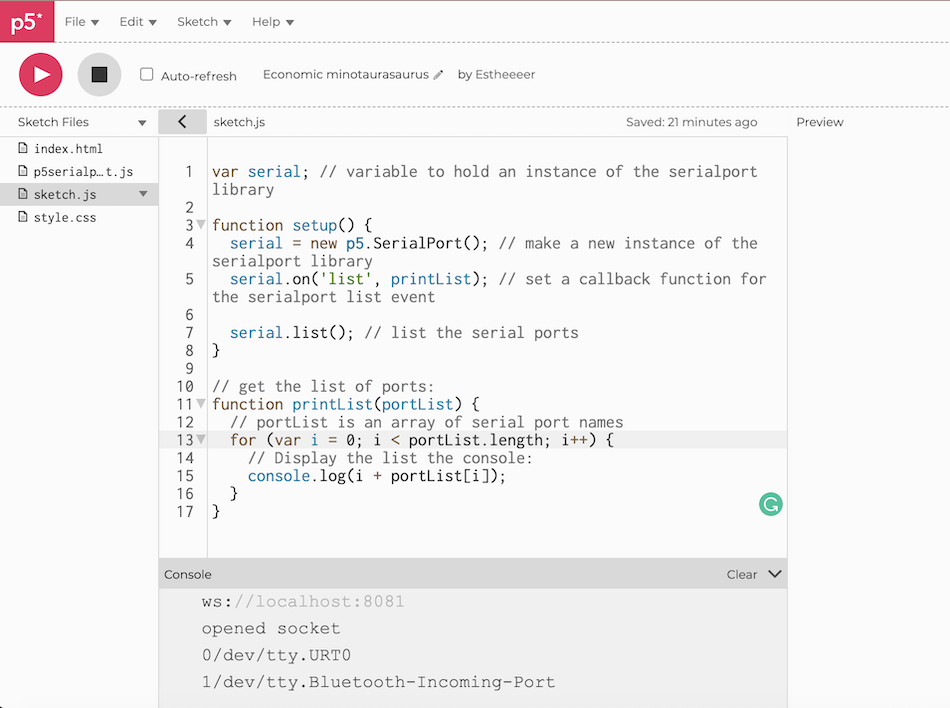
Call back function
set up a call back function
serial.on('list', printList);
Call the function with the given name above in ‘ ‘ (so here, the ‘list’ become one trait of the object serial)
serial.list();
Main body of the function:
// get the list of ports:
function printList(portList) {
// portList is an array of serial port names
for (var i = 0; i < portList.length; i++) {
// Display the list the console:
console.log(i + portList[i]);
}
}
In p5.js, data rate: 9600 bits per second is the default and don’t need to add it to the code, unless we want to set different rate.
I tried to apply the example that showing potentiometer value in the browser, but I got “sensor value: undefined” instead. I checked that my web socket and serial port were both connected successfully. I am not sure why this happened.
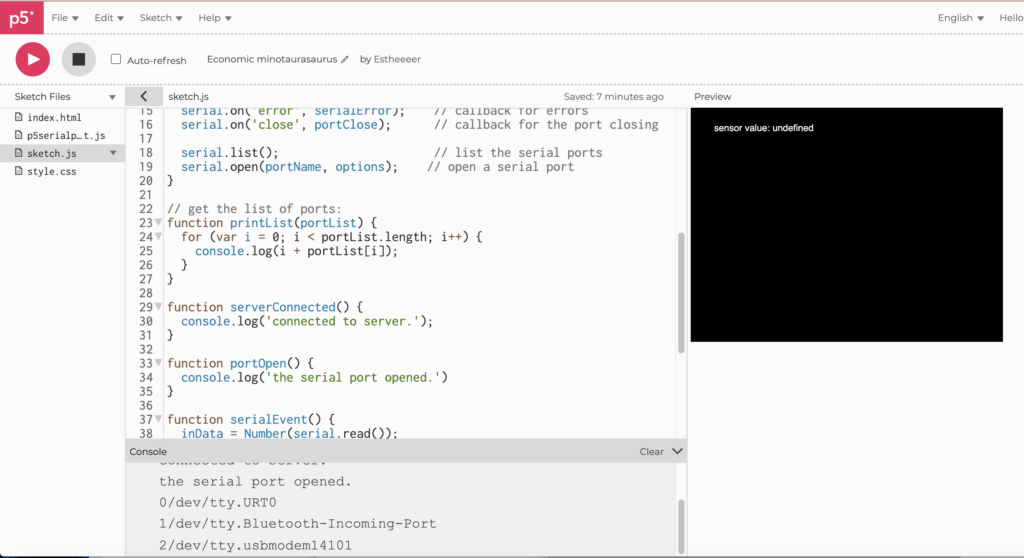
After rechecking my P5.serialControl API, I found that several ports were listed and open, which might cause the problem. I cleared out all the ports and reconnected everything, values showed in the browser but with a very fast speed.
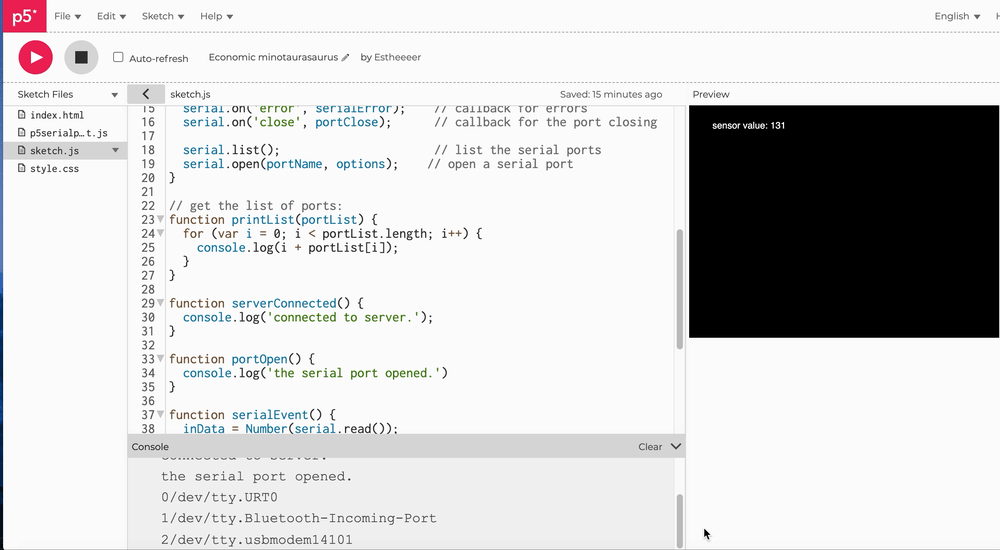
Some questions
Q: Is this the only way to disconnect program with the serial port?
Q: Why I can’t install coolterm? – Fixed. Change the preference of security on my mac pro.
Q: I am not quite understand the Flow control example.